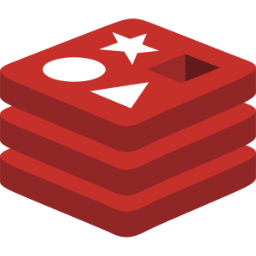
const classredis::RedisClient
sys::Obj redis::RedisClient
Redis client.
- close
Void close()
Close this client all connections if applicable.
- del
Delete the given key value.
- expire
Void expire(Str key, Duration seconds)
Expire given key after given
seconds
has elasped, where timeout must be in even second intervals.- expireat
Void expireat(Str key, DateTime timestamp)
Expire given key when the given
timestamp
has been reached, wheretimestamp
has a resolution of whole seconds.- get
Get the value for given key.
- hdel
Delete given hash field for key.
- hget
Get the hash field for given key.
- hgetall
Get all hash field values for given key.
- hincr
Convenience for
hincrby(key, field 1)
- hincrby
Int hincrby(Str key, Str field, Int delta)
Increments the number stored at
field
in the hash stored atkey
by givendelta
. If the field does not exist, it is set to 0 before performing the operation.- hincrbyfloat
Float hincrbyfloat(Str key, Str field, Float delta)
Increment the string representing a floating point number stored at
field
in the hash stored atkey
by the specifieddelta
. If the key does not exist, it is set to 0 before performing the operation.- hmget
Str?[] hmget(Str key, Str[] fields)
Get the hash field for given key.
- hmset
Void hmset(Str key, Str:Obj vals)
Set all hash values in
vals
for given key.- host
const Str host
Host name of Redis server.
- hset
Void hset(Str key, Str field, Obj val)
Set the hash field to the given value for key.
- incr
Int incr(Str key, Duration? px := null)
Increments the number stored at key by one. If the key does not exist, it is set to 0 before performing the operation. If
px
is non-null expire this key after the given timeout in milliseconds. Returns the value of the key after the increment.- incrby
Int incrby(Str key, Int delta, Duration? px := null)
Increments the number stored at key by
delta
. If the key does not exist, it is set to 0 before performing the operation. Ifpx
is non-null expire this key after the given timeout in milliseconds. Returns the value of the key after the increment.- incrbyfloat
Float incrbyfloat(Str key, Float delta, Duration? px := null)
Increment the string representing a floating point number stored at
key
by the specifieddelta
. If the key does not exist, it is set to 0 before performing the operation. Ifpx
is non-null expire this key after the given timeout in milliseconds. Returns the value of the key after the increment.- invoke
Invoke the given command and return response.
- make
new make(Str host, Int port := 6379)
Create a new client instance for given host and port.
- memStats
Returns information about the memory usage of server.
- pexpire
Void pexpire(Str key, Duration milliseconds)
Expire given key after given
ms
has elasped, where timeout must be in even millisecond intervals.- pipeline
Obj?[] pipeline(Obj[] invokes)
Pipeline multiple invoke requests and return batched results.
- port
const Int port
Port number of Redis server.
- set
Void set(Str key, Obj? val, Duration? px := null)
Set the given key to value, if
val
is null this method deletes the given key (see del). Ifpx
is non-null expire this key after the given timeout in milliseconds.- setnx
Bool setnx(Str key, Obj val, Duration? px := null)
Set the given key to value only if key does not exist. Returns
true
if set was succesfull, or false if set failed due to already existing key. Ifpx
is non-null expire this key after the given timeout in milliseconds.